Embedding a Github Calendar in a React App
Embedding a Github Calendar into a React app is a fairly straight forward process. I used react-github-calendar to do this.
First, install the package:
npm install react-github-calendar
or with
yarn
useyarn add react-github-calendar
Then, import the component and use it in your app:
import GitHubCalendar from 'react-github-calendar'
const MyCalendar = () => {
return <GitHubCalendar username="patebry" />
}
export default MyCalendar
You can easily update the
username
prop to display your own Github calendar.
You should see a calendar that looks like this:
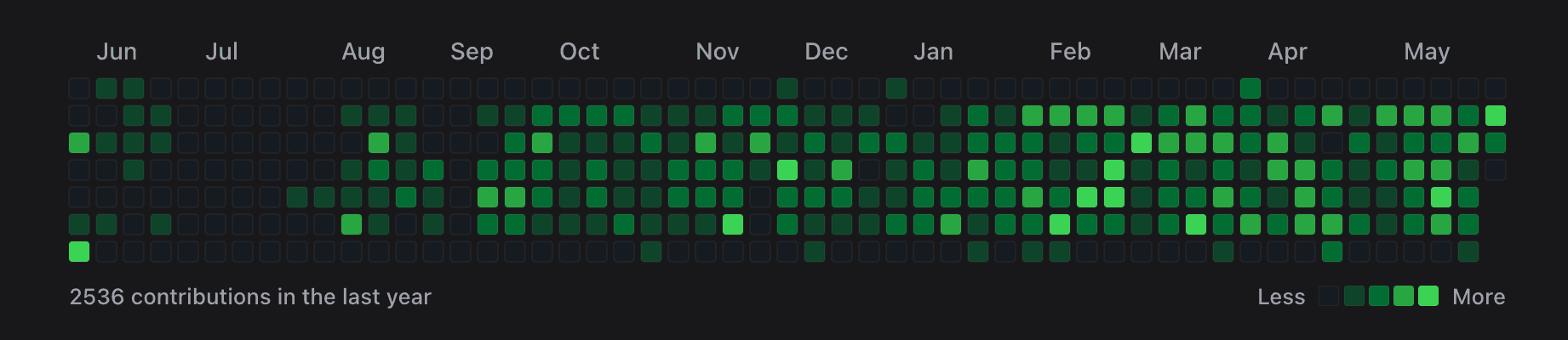
It's also easy to update the theme of the calendar with the following code:
import GitHubCalendar from 'react-github-calendar'
const MyCalendar = () => {
return (
<GitHubCalendar
username="patebry"
colorScheme="light"
theme={{
light: [
'rgba(45, 212, 191, 0.0)',
'rgba(45, 212, 191, 0.4)',
'rgba(45, 212, 191, 0.6)',
'rgba(45, 212, 191, 0.8)',
'rgba(45, 212, 191, 1)',
],
}}
/>
)
}
export default MyCalendar
This is what my site looks like with the calendar embedded:
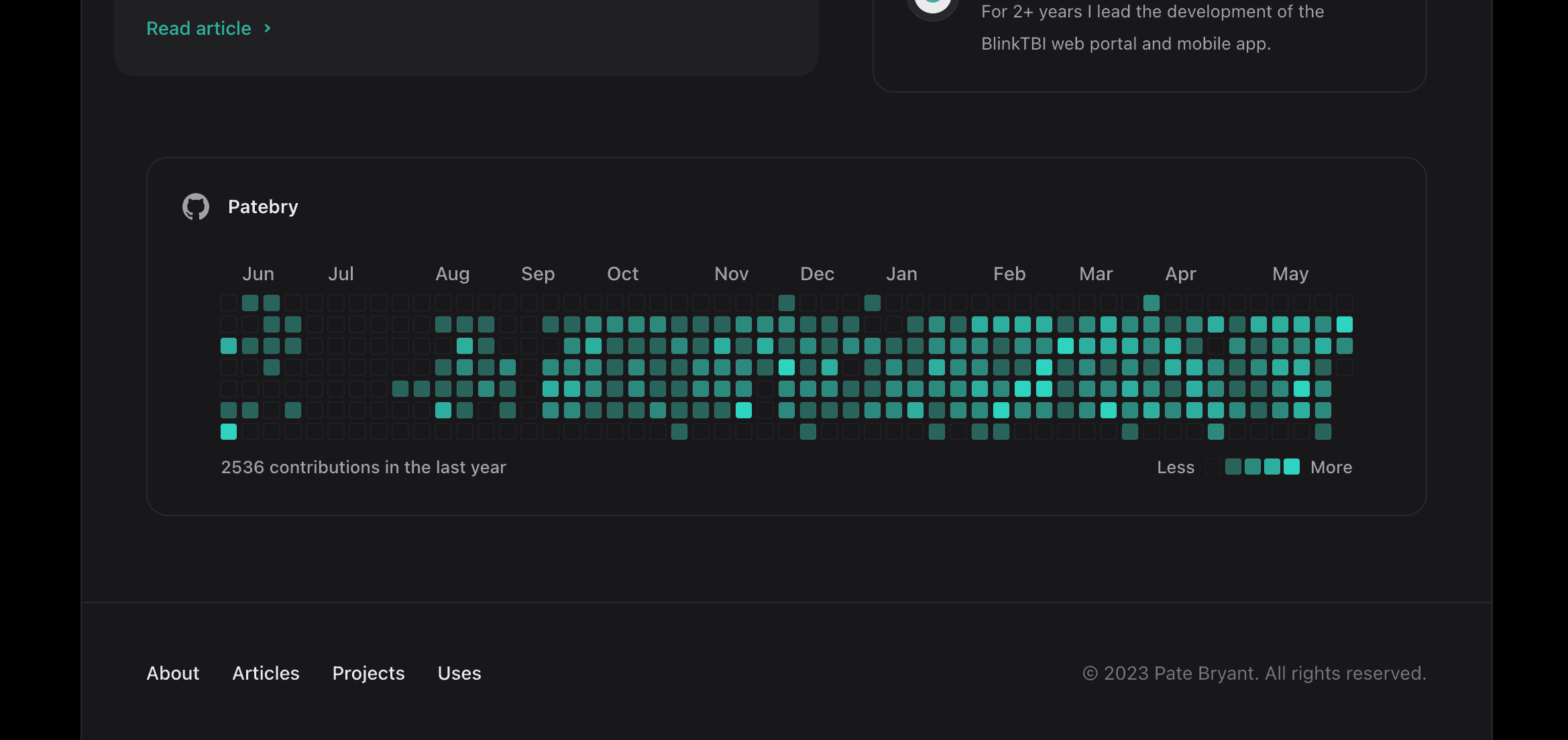
You can see this calendar live here .